Python has become one of the most famous programming dialects because of its effortlessness, flexibility, and strong abilities. Whether you are a fledgling or an accomplished engineer, dominating Python can extraordinarily upgrade your programming abilities. In this article, we will investigate ten vital Python tips that will assist you with composing more effective, clear, and viable code. From upgrading execution to taking care of special cases, these tips will support your efficiency and further develop your general programming experience.
Table of Contents:
1. Use List Comprehensions |
2. Leverage Generators |
3. Employ Context Managers |
4. Understand Decorators |
5. Handle Exceptions Gracefully |
6. Optimize Performance with Libraries |
7. Utilize Object-Oriented Programming |
8. Document Your Code |
9. Use Virtual Environments |
10. Collaborate with Version Control |
1. Use List Comprehensions
Python’s rundown perceptions give a succinct and proficient method for making records in light of existing records or other iterable items. By utilizing list understandings, you can compose minimized code that performs activities, for example, sifting, planning, and changing components. This outcomes in cleaner and more meaningful code.
List understandings follow the sentence structure: [expression for thing in iterable if condition]. The articulation is assessed for every thing in the iterable, and the subsequent components are gathered in another rundown. We should think about a model:(python tips)
Example: Squaring numbers using list comprehension
numbers = [1, 2, 3, 4, 5]
squared_numbers = [num ** 2 for num in numbers]
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
2. Leverage Generators
Generators are a significant device in Python for making iterators. Not at all like records, generators produce values on-the-fly, which can save memory and further develop execution, particularly while managing enormous datasets. Generators utilize the yield watchword to return esteems each in turn.
To make a generator, you characterize a capability with the yield proclamation. Each time the generator is iterated, it executes the capability until it arrives at a yield explanation, which returns a worth. The capability’s state is then saved, and it resumes execution when the following worth is mentioned. Here is a model:(python tips)
Example: Fibonacci sequence generator
def fibonacci():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
fib_gen = fibonacci()
1.print(next(fib_gen)) # Output: 0
2.print(next(fib_gen)) # Output: 1
3.print(next(fib_gen)) # Output: 1
4.print(next(fib_gen)) # Output: 2
3. Employ Context Managers
Setting supervisors assist with guaranteeing that assets are appropriately made due, particularly while managing records, network associations, or information base exchanges. Python furnishes a helpful method for working with setting directors utilizing the with proclamation.
By utilizing the with articulation, you can ensure that the assets are consequently delivered after their utilization, regardless of whether exemptions happen. The setting supervisor’s enter strategy is called while entering the block and exit is called while leaving the block. Here is a model:(python tips)
Example: Opening a file using a context manager
with open(‘data.txt’, ‘r’) as file:
content = file.read()
print(content)
The file is automatically closed outside the ‘with’ block
4. Understand Decorators
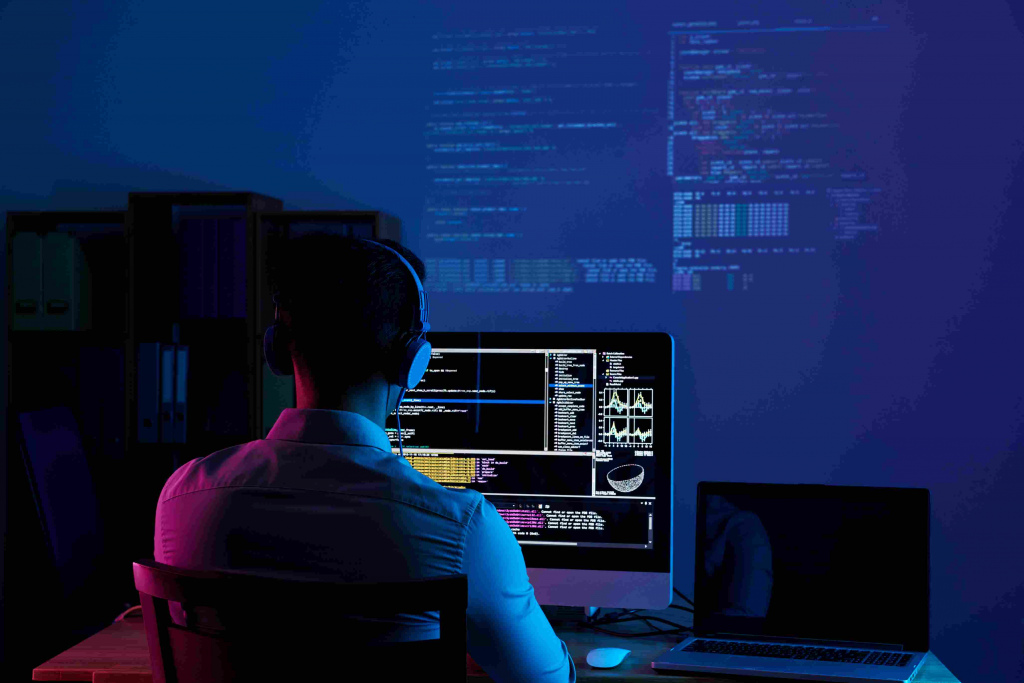
Decorators permit you to alter the way of behaving of capabilities or classes without changing their source code. They give a perfect and adaptable method for adding usefulness like logging, timing, or access control to existing capabilities.
A decorator is a capability that accepts one more capability as information and returns a changed capability. It wraps the first capability, permitting you to execute extra code previously or potentially after its execution. This can be valuable for executing cross-cutting worries. Here is a model:(ptyhon tips)
Example: Simple logging decorator
def log_decorator(func):
def wrapper(*args, *kwargs): print(f”Calling {func.name}…”) result = func(args, **kwargs)
print(f”{func.name} called.”)
return result
return wrapper
@log_decorator
def say_hello(name):
print(f”Hello, {name}!”)
say_hello(“John”)
#Output:
#Calling say_hello…
#Hello, John!
#say_hello called.
5. Handle Exceptions Gracefully – Python
Special case dealing with is significant for composing powerful and dependable code. Python gives a thorough component to dealing with exemptions, permitting you to smoothly recuperate from mistakes and keep your program from crashing.
To deal with special cases, you utilize an attempt aside from block. The code that might actually raise a special case is put inside the attempt block. Assuming an exemption happens, it is gotten by the comparing aside from block, permitting you to deal with the mistake or make a fitting move. Here is a model:(python tips)
Example: Handling division by zero
try:
result = 10 / 0
except ZeroDivisionError:
print(“Cannot divide by zero!”)
6. Enhance Execution with Python Libraries
Python offers an immense environment of libraries that can incredibly upgrade your code’s presentation. By utilizing these libraries, you can offload computationally escalated assignments to profoundly advanced C or Fortran code, bringing about huge speed enhancements.
A few famous libraries for execution enhancement incorporate NumPy, which gives productive exhibit tasks, and Pandas, which offers strong information control capacities. Also, devices like Cython permit you to compose Python code that can be assembled to C, further supporting execution.
7. Use Item Situated python Programming
Python upholds object-arranged programming (OOP), permitting you to coordinate your code into reusable and secluded parts. By characterizing classes and making objects, you can typify information and conduct, making your code more organized and simpler to keep up with.
OOP empowers ideas like legacy, polymorphism, and exemplification, giving strong ways of displaying complex frameworks. It advances code reusability, measured quality, and division of worries. Understanding and applying OOP standards can extraordinarily improve your Python programming abilities.
8. Record Your Python Code
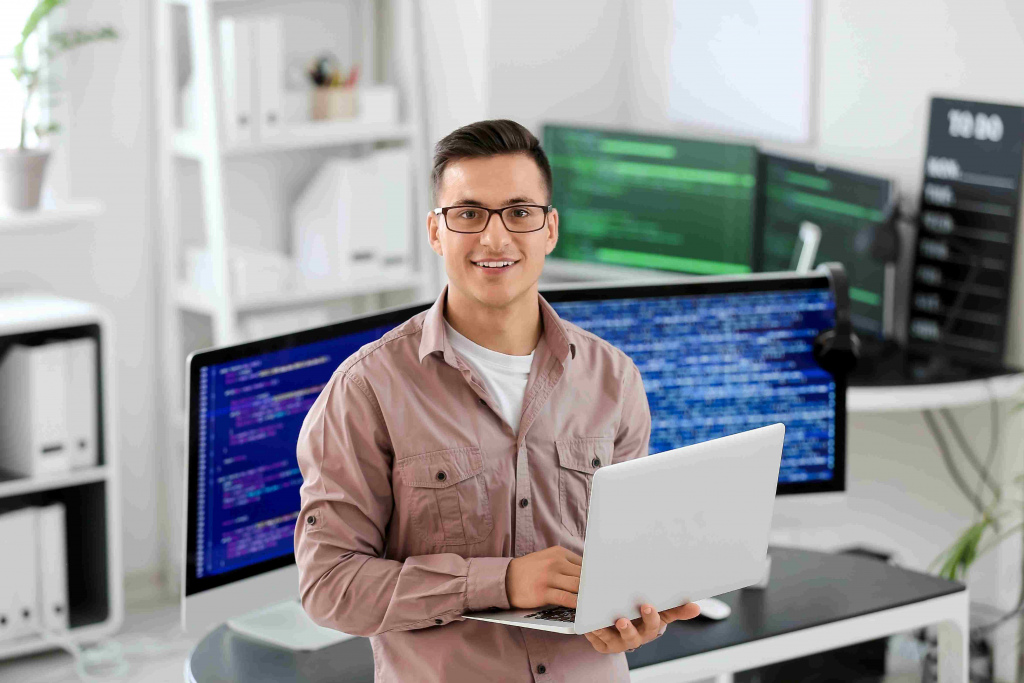
Appropriate documentation is fundamental for keeping up with and working together on code projects. Python has an underlying documentation structure called docstrings, which permits you to record your code, capabilities, classes, and modules.
By giving clear and brief clarifications, models, and use directions in your docstrings, you make it more straightforward for other people (and yourself) to comprehend and work with your code. Besides, many devices and IDEs can consequently create documentation from very much organized docstrings, working with code investigation and reuse.
9. Utilize Virtual Conditions
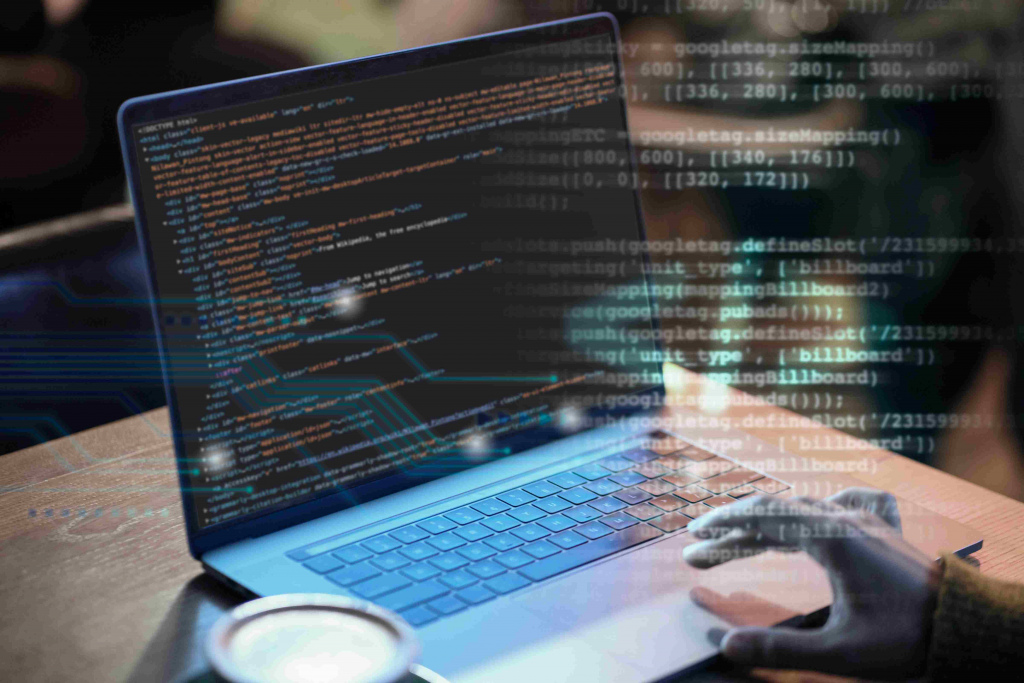
Virtual conditions empower you to establish disconnected Python conditions for various tasks or applications. They assist with overseeing conditions, guaranteeing that each task utilizes the particular renditions of libraries it expects, without clashes with different undertakings.
By utilizing virtual conditions, you can stay away from the traps of reliance damnation and guarantee reproducibility across various advancement conditions. Python gives instruments like ‘venv’ and ‘conda’ for establishing and overseeing virtual conditions
10. Work together with Adaptation Control
Rendition control frameworks like Git give a strong method for working together on code projects, track changes, and oversee various variants of your codebase. By utilizing variant control, you can work consistently with groups, roll back to past forms, and effectively combine changes.
Utilizing Git, you can make storehouses, commit changes, branch out for highlight improvement, and union branches once again into the fundamental codebase. Facilitating stages like GitHub, GitLab, or Bitbucket give extra highlights to give following, code surveys, and nonstop incorporation.
Every now and again Got clarification on some things (FAQs)
Q: Is Python a decent programming language for novices?
A: Indeed, Python is viewed as one of the most incredible programming dialects for novices because of its basic language structure and lucidness.
Q: How might I further develop my Python programming abilities?
A: Practice routinely, investigate Python libraries, read code from experienced designers, and take part in coding difficulties and undertakings.
Q: What is the reason for virtual conditions?
A: Virtual conditions permit you to establish disengaged Python conditions to oversee conditions and guarantee reproducibility of undertakings.
Q: Might I at any point involve Python for web advancement?
A: Indeed, Python is broadly utilized for web improvement. Structures like Django and Carafe give strong apparatuses to building web applications.
Q: How might I enhance the presentation of my Python code?
A: Use libraries like NumPy and Pandas for productive information handling, profile your code to recognize bottlenecks, and think about utilizing ordered expansions.
Q: What is the meaning of reporting code?
A: Legitimate documentation further develops code comprehensibility, works with coordinated effort, and permits others to really comprehend and utilize your code.
Q: Might I at any point involve Python for AI and information examination?
A: Totally! Python has a rich environment of libraries, including scikit-learn and TensorFlow, that pursue it a well known decision for AI and information investigation.
Q: What are a few prescribed procedures for exemption taking care of in Python?
A: Catch explicit exemptions as opposed to utilizing a conventional with the exception of block, handle special cases at a proper level, and give educational blunder messages.
Q: Is object-situated programming vital in Python?
A: Article situated writing computer programs isn’t completely vital, yet it offers strong ways of sorting out and structure code, especially for complex ventures.
Q: How could form control frameworks benefit my Python projects?
A: Rendition control frameworks like Git consider cooperative turn of events, simple following of changes, and consistent converging of code commitments.
End:
Dominating Python requires ceaseless learning and practice. By following these ten vital hints, you can fundamentally improve your Python programming abilities and compose more compelling code. Make sure to use list appreciations and generators for compact and effective code, utilize setting directors and decorators for better asset the executives and code customization, handle special cases nimbly to guarantee vigor, and investigate libraries for execution streamlining. Moreover, embrace object-arranged programming, report your code successfully, utilize virtual conditions for reliance the executives, and team up with variant control frameworks. By applying these tips, you’ll turn into a more capable Python software engineer, equipped for handling different programming difficulties easily.